Your First Pull Request
Finding a bug in an open source software library or application is frustrating. Finding the error and coming up with a solution is exciting. Submitting a pull request (PR) to the maintainer is daunting.
Setup GitHub
Chances are good that if you are working with an open source project they've hosted it on GitHub. The first thing to do is get yourself a free GitHub account.
Fork the Repository
Go to the GitHub page for the project you want to fix. Once there click the <Fork> button.
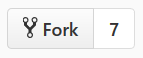
This will give you a copy of the entire source code where you can fix the bugs and run the tests. You will be automatically redirected to your new copy of the repo. You'll notice that it shows you the project your forked from and that you have an exact copy of everything, including the entire history of changes made to the code.
Install Git
You'll need Git installed on your computer to track and commit the changes you make to any of the code. Depending on your OS you may already have it installed. If not, download and install it.
Clone the Repo
Now that you have a copy of the code in your own repo, you need a copy of the repo on your computer where you can edit it, run it, and test it. To do that we need to clone the repository.
First create an empty directory where we'll clone your repository.
In your command prompt or terminal navigate into the empty folder you created and clone your repository:
git clone https://github.com/your_github_username/your_repository_name.git
You'll see something like this:
Cloning into 'your_repo_name'... remote: Counting objects: 1926, done. remote: Compressing objects: 100% (68/68), done. remote: Total 1926 (delta 37), reused 0 (delta 0), pack-reused 1858 Receiving objects: 100% (1926/1926), 1.59 MiB | 667.00 KiB/s, done. Resolving deltas: 100% (1164/1164), done.
If you haven't yet you will need to set some basic info into your git config. You can't commit changes until you do.
git config --global user.email "your_email" git config --global user.name "your name"
Make The Fix
Now that you have a local copy of the code you can begin editing it. Go ahead and make the changes you need to make and test those changes. Testing may be as simple as manually checking the thing you noticed was broken, but it may also include running the project's test suite. You may also need to write a few tests to make sure that the thing you just fixed is not broken in future releases. Doing that depends a great deal on the language and testing framework being used. If you aren't sure, reach out to the project maintainer for guidance.
With the code (and possibly test) changes made you need to commit your changes and then push them to the repository you created on GitHub.
Start by adding the files you edited or created. For each file you added or changed run this command.
git add your_file_name
Next commit the changes you've staged. Add a meaningful message that describes the change.
git commit -m "Your commit message goes here"
Now we are ready to push the changes from your local repository to your remote repository on GitHub. You may be asked for your username and password. Enter those at the prompts.
git push https://github.com/your_github_username/your_repository_name.git
Issue a Pull Request
A pull request is a way of asking the project maintainer to add your code changes to the master repository that others will work from and use. It lets you pick just the change set you want.
From GitHub open the project you want where you want your code included. Click the Pull Requests tab and then click the New pull request button.

Because you want to have your code changes included in another project click the link for 'compare across forks'. This will give you the chance to pick both the original repository your forked from and your repository with changes.

In the base fork pick the project in which you want to include your code change. Chances are you want it in the master fork. For head fork pick your repository and compare against the master. With any luck you'll see that you are 'Able to merge'. This means that you don't have any code changes that conflict with code changes made by someone else in the time between you forking the repository and filing your pull request. It doesn't mean there are guaranteed not to be any problems, but it does mean that you didn't change the same code as someone else.

Now you are ready to finalize your request. Start by clicking Create pull request. Add some information to give the person reviewing your request an explanation as to what you were fixing and how you fixed it. Once you've given the maintainer as much info as you can click Create pull request.
What Happens Now
At this point the project maintainer will get notified that a pull request has been created. They will see the changes you made and can review them to make sure they want to include them in the source code.
In addition to manual review, your version of the code may be run through something like Travis CI. Continuous integration tools like this will run automated tests to determine if your change breaks anything. It may test the code on multiple platforms or multiple versions of programming language looking for problems.
Four things can happen next:
Acceptance
The maintainer may accept and merge your pull request. This might even happen automatically if your change passes the continuous integration testing.
Feedback
Or they may come back to you with questions or comments. They might request that you change the code you submitted. They might want you to follow a specific style or make the change elsewhere in the code base. Perhaps they require documentation to go along with the change you made.
Rejection
Or they may reject your pull request. It might be that you tried to add a feature they don't want included. Or they may want more thorough unit tests. It could be that while you really did find a bug, that bug has now become a 'feature' and fixing it would cause problems to users they aren't ready to deal with yet. If your pull request is rejected it is okay to start a friendly conversation to find out why. You can always continue using your code in your projects.
Nothing
Finally, they may do nothing. Remember that as a rule the maintainer of the project you are trying to fix isn't getting paid to do this work. They have busy lives and other obligations. Be patient.